First, before fixing that famous error, we want to define and go a little in-depth with router-outlet, the definition why we use it, the error of router-outlet is not a known element! and how to fix it.
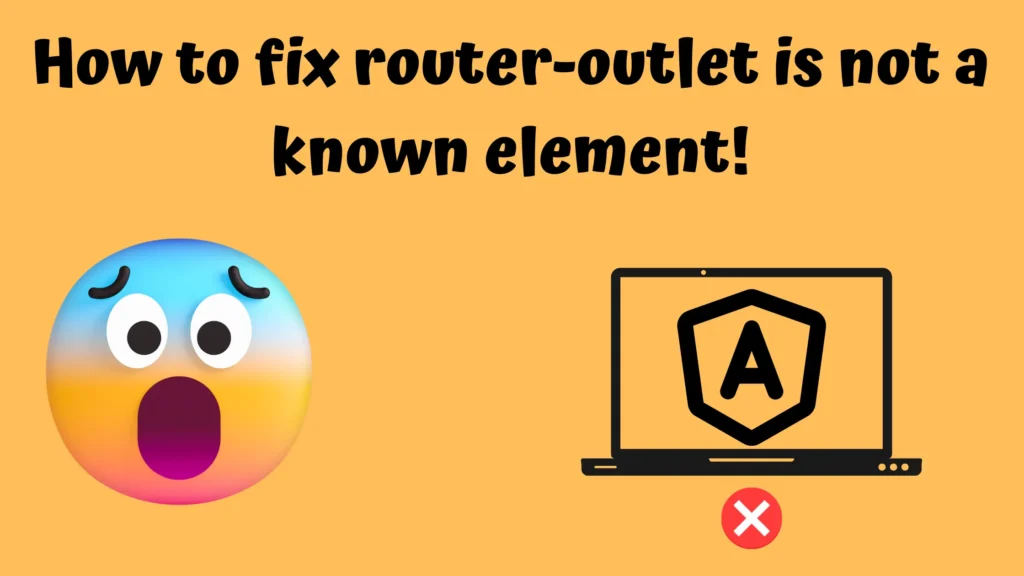
What is router-outlet (Routing outlet) in angular?
router outlet or router-outlet is a built-in directive in Angular that helps choose the appropriate component to display on the screen.
router-outlet is not a known element
Now, we will move to the famous error. While adding the router outlet directive in the needed HTML emplacement, sometimes you may encounter this error, telling you that router-outlet is not a known element.
let’s take a look at how to add a router outlet directive.
//app.component.html
<router-outlet></router-outlet>
In that way, Angular will be responsible to take the active route and displaying the appropriate component. But, Sometimes an error in the console may appear telling that “router-outlet is not a known element“.
The causes behind this error are multiple. So we will choose the repetitive ones.
Not importing the RouterModule which is responsible for calling the router outlet.
Be careful, if you have a separate routing file you must declare the RouterModule and exports it, or import it directly into the app module file.
This error may occur while working with lazy loading modules , So be wise when you take the solution provided below.
Solution :
//app-routing.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { RouterModule, Routes } from '@angular/router';
import { ProductListComponent } from './product-list/product-list.component';
const routes: Routes = [
{path:"products",component:ProductListComponent}
]
@NgModule({
declarations: [],
imports: [
CommonModule,
RouterModule.forRoot(routes)
],
exports: [ RouterModule ]
})
export class AppRoutingModule { }
//app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { AppRoutingModule } from './app-routing.module';
@NgModule({
declarations: [
AppComponent,
],
imports: [
BrowserModule,
AppRoutingModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
router outlet is not a known element unit test
Yes, this error may appear while working in unit testing.
Solution
You should so import RouterTestingModule into your app.component.spec.ts like this :
//app.component.spec.ts
import { TestBed } from '@angular/core/testing';
import { AppComponent } from './app.component';
import { RouterTestingModule } from '@angular/router/testing';
describe('AppComponent', () => {
beforeEach(async () => {
await TestBed.configureTestingModule({
imports: [
RouterTestingModule
],
declarations: [
AppComponent
],
}).compileComponents();
});
it('should create the app', () => {
const fixture = TestBed.createComponent(AppComponent);
const app = fixture.componentInstance;
expect(app).toBeTruthy();
});
it(`should have as title 'Ecommerce-Project'`, () => {
const fixture = TestBed.createComponent(AppComponent);
const app = fixture.componentInstance;
expect(app.title).toEqual('Ecommerce-Project');
});
it('should render title', () => {
const fixture = TestBed.createComponent(AppComponent);
fixture.detectChanges();
const compiled = fixture.nativeElement as HTMLElement;
expect(compiled.querySelector('.content span')?.textContent).toContain('Ecommerce-Project app is running!');
});
});
Conclusion
There are many reasons behind getting router-outlet is not a known element and we tried to cover the most common cases and the ways to solve them, but generally, it’s by importing the RouterModule.