For some reasons, you want to determine the active route in Angular project. That’s why, the powerful Angular Router implements some built-in classes, that help us easily get the current route path.
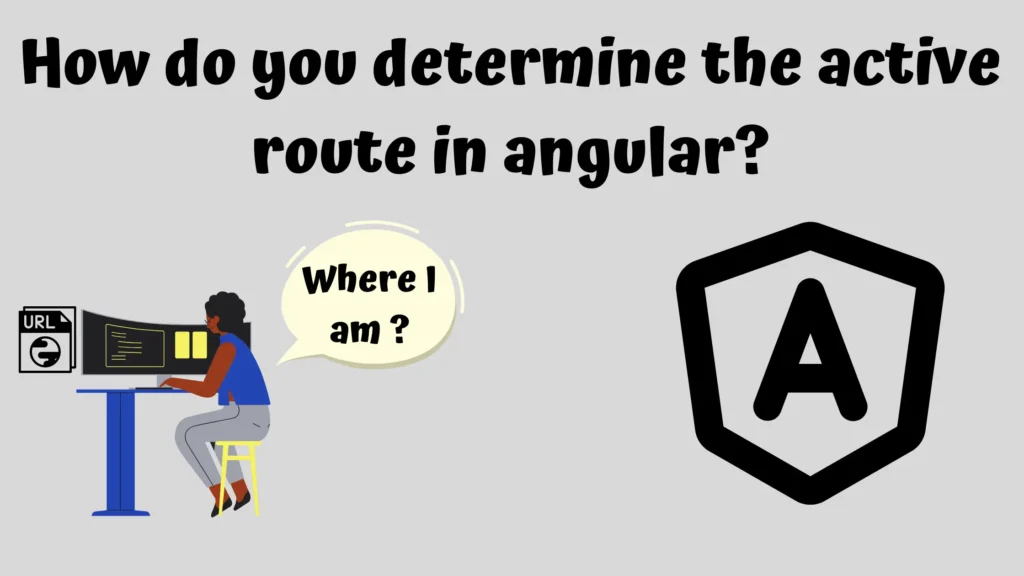
How do you determine the active route in angular?
The steps are too easy :
- Import Router,NavigationEnd from ‘@angular/router’ and inject the first in the constructor.
- Subscribe to the NavigationEnd event of the router.
- Get the current route url by accessing NavigationEnd’s url property.
let’s make those steps into readable code.
//app.component.ts
import { Component } from '@angular/core';
import { Router,NavigationEnd } from '@angular/router';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
title = 'Ecommerce-Project';
currentRoute: string;
constructor(private router: Router){
router.events.filter(event => event instanceof NavigationEnd)
.subscribe(event =>
{
this.currentRoute = event.url;
console.log(event);
});
}
}
We’ve created the currentRoute variable to track the variation of the current route, and we will see that in the interface in a realtime. Let’s see How .
//app.component.html
<ul>
<li routerLinkActive="active">
<a [routerLink]="['/about']">About</a>
</li>
<li routerLinkActive="active">
<a [routerLink]="['/addProduct']">Add Product</a>
</li>
<li routerLinkActive="active">
<a [routerLink]="['/productList']">Product List</a>
</li>
</ul>
{{currentRoute}}
<router-outlet></router-outlet>
We have 3 routes in this example which are about, addProduct, and productList. By navigating from one route to another, we will see that currentRoute change its value every time by interpolation.
Conclusion
In this blog post, we discovered how we can get the current route in Angular, We can do it with Angular Router API.
Stay Tuned and look at our blog posts.