We consisted on Why you should learn Angular? Everything you need to know on the obligation of acquiring some minimal knowledge before starting learning Angular. So, if you are familiar with Javascript you evidently know how to use javascript forEach(). But, Suppose that we are having a repetitive HTML block that must contain different data. Are we obliged to repeat each HTML block with their Data?
Angular has helped us by predefining a structural Directive called ngFor directive.
Stay with us to know :
- Why ngFor is used in Angular?
- How does ngFor work in Angular with examples?
- Common questions about ngFor.
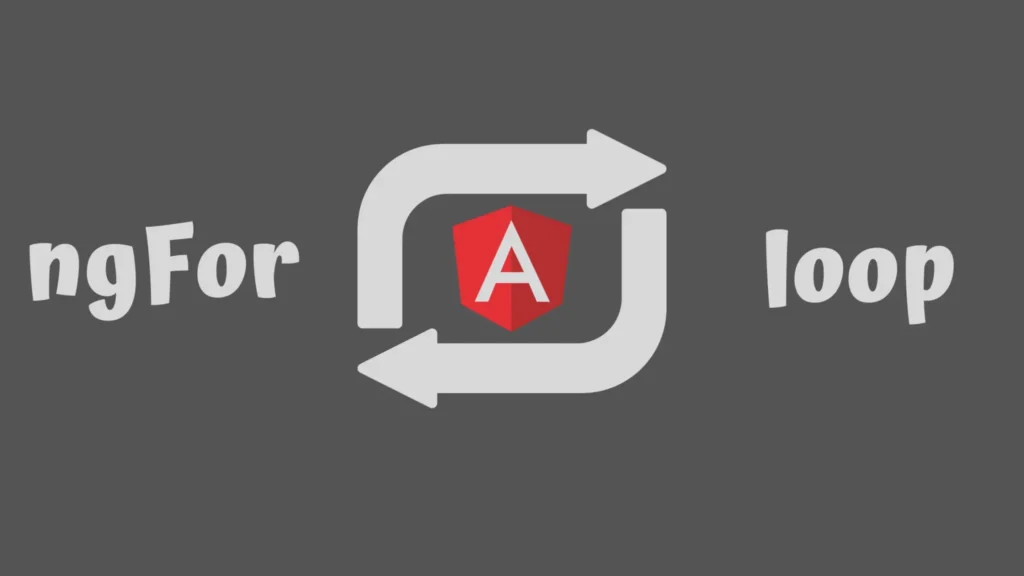
Why ngFor is used in Angular?
As described above, Angular helped us to create HTML elements and blocks as many times as the Array length ( elements in the Array ), by providing the structural directive *ngFor.
It facilitates looping over HTML blocks.
How does ngFor work in Angular with examples?
You may ask how does ngFor work? Don’t worry my friend. We will help you by giving a real example of how this Angular HTML looping directive.
Syntax of ngFor
We said that ngFor is used to handle Arrays and to loop over its elements to create HTML elements.
<ul *ngFor="let product of Products">
<li>
...
</li>
</ul>
We will explain the code written above in the next part of the *ngFor example.
ngFor Example
In this section, we will get into depth with a real use case of ngFor.
Hence, in order to avoid repeating each HTML portion like the example below,
//product-list.component.html
<ul>
<li>
product1
<!-- id of the first product -->
</li>
</ul>
<ul>
<li>
product2
<!-- id of the second product -->
</li>
</ul>
<ul>
<li>
product3
<!-- id of the third product -->
</li>
</ul>
We will use ngFor to reduce the code written and to simplify the treatment.
//product-list.component.html
<ul *ngFor="let product of products">
<li>
{{product.label}}
</li>
</ul>
and in the Typescript file, we must have the Products Array containing all the elements that we want to display dynamically in the ul element.
//product-list.component.ts
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-product-list',
templateUrl: './product-list.component.html',
styleUrls: ['./product-list.component.css']
})
export class ProductListComponent implements OnInit {
Products = [
{ Id: 1, label: 'Iphone 13 Pro Max' },
{ Id: 2, label: 'Iphone 12 Pro Max' },
{ Id: 3, label: 'Iphone 11 Pro Max' }
];
constructor() { }
ngOnInit(): void
{
}
}
Local Variables of ngFor Directive
When working with ngFor you may need some predefined variables to get some required information like the index of the element that we are looping over. Angular made this task easy too, by providing a lot of variables combined with the ngFor Directive called Local Variables of ngFor Directive.
- index: number: The index of the current element in the Array.
- count: number: The length of the Array.
- first: boolean: True when the element is the first item in the Array.
- last: boolean: True when the element is the last item in the Array.
- even: boolean: True when the element has an even index in the Array.
- odd: boolean: True when the element has an odd index in the Array.
The variables listed above are called locally because they can be used only in the HTML tag in which we defined the ngFor or ints children.
Here you can find a simple example of the index odd, and even variables.
//product-list.component.html
<div *ngFor="let product of products;
let isOdd=odd;
let isEven=even"
[class.odd]="isOdd"
[class.even]="isEven" >
<p>{{product.label}}</p>
</div>
trackBy with ngFor
You may be asking how Angular will handle changes in the Array that we are looping over. We are talking about adding Items or removing them. Angular tries in that way to create or remove HTML elements ( It depends on the case ). It means that we are facing complex DOM manipulations, especially in the case of A big Array.
For such cases, and to boost the application performance ( by reducing expensive DOM manipulations) we can supply the trackBy function which will help by acting only on the items that are modified ( added or removed ).
you can see the example above to understand more.
@Component({
selector: 'app-product-list',
template: `
<ul>
<li *ngFor="let product of Products;trackBy: trackByFn">{{product.label}} </li>
</ul>
<button (click)="getProducts()">Refresh products</button>
`,
})
export class App {
constructor() {
Products = [
{ Id: 1, label: 'Iphone 13 Pro Max' },
{ Id: 2, label: 'Iphone 12 Pro Max' },
{ Id: 3, label: 'Iphone 11 Pro Max' }
]; }
getProducts() {
this.Products = this.getItemsFromServer();
}
getItemsFromServer() {
return
{ Id: 1, label: 'Iphone 13 Pro Max' },
{ Id: 2, label: 'Iphone 12 Pro Max' },
{ Id: 3, label: 'Iphone 11 Pro Max' },
{ Id: 4, label: 'Iphone 11 Pro ' },
];
}
trackByFn(index, item) {
return index;
}
}
At this level, we think that we have covered a lot of ngFor features.
Conclusion
To summarize, We have covered the purpose of using ngFor Directive with some examples. Also, We have discovered together with some advanced functions and variables like trackBy, index, odd, even, and others …