If you’re an Angular developer, you may have encountered the infamous “Can’t bind to ‘ngModel'” error. This error message can be frustrating and confusing, especially if you’re new to Angular. However, there is a simple solution to this error that can save you a lot of time and effort. In this blog post, we’ll walk you through how to fix the “Can’t bind to ‘ngModel'” error in Angular.
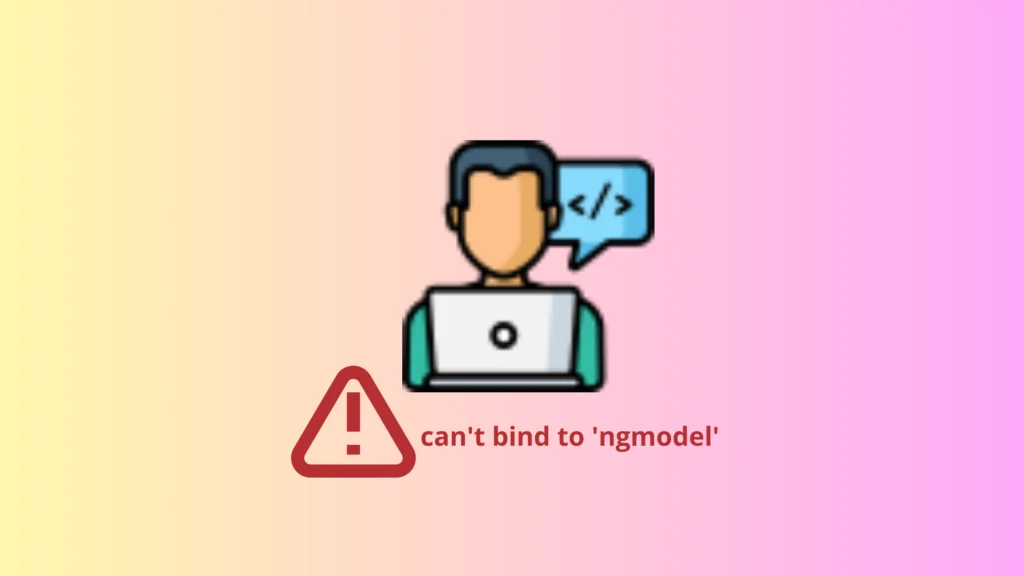
How to fix “Can’t Bind to ‘ngModel'” Error in Angular:
Step 1: Import FormsModule
The first step to fixing the “Can’t bind to ‘ngModel'” error is to ensure that the FormsModule is imported in your Angular module. The FormsModule is a module that provides support for two-way data binding using the ngModel directive.
To import the FormsModule, simply add it to the imports array in your app.module.ts file:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Step 2: Use the ngModel Directive
The next step is to use the ngModel directive in your HTML template. The ngModel directive is used to bind an input, select, or textarea element to a property on the component.
To use ngModel, simply add it to your input element:
In the above example, we’re using ngModel to bind the value of the input element to a “name” property on our component. This will allow us to update the “name” property whenever the input value changes, and vice versa.
Step 3: Use the Name Property
The final step is to ensure that the “name” property is declared on our component. This can be done by adding a “name” property to the component class:
export class AppComponent {
name: string = '';
}
In the above example, we’re declaring a “name” property on our component with an initial value of an empty string. This will allow us to store the value of the input element and use it elsewhere in our component.
Code Snippet Example:
Here’s an example code snippet that implements the above steps:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: <h1>Hello {{ name }}!</h1> <input [(ngModel)]="name">
})
export class AppComponent {
name: string = '';
}
Conclusion
The “Can’t bind to ‘ngModel'” error is a common error in Angular, but it’s also an easy error to fix. By following the steps outlined in this blog post, you can quickly resolve this error and continue building your Angular application with ease.
If you found this blog post helpful, be sure to follow our blog for more useful tips and tricks on Angular development. Happy coding!