Introduction
If you are an Angular developer, you must have come across an error message that reads “Expression has changed after it was checked”. The error message can be frustrating, and you may not know where to start in fixing it. In this blog post, I will guide you through the steps to solve this error in Angular.
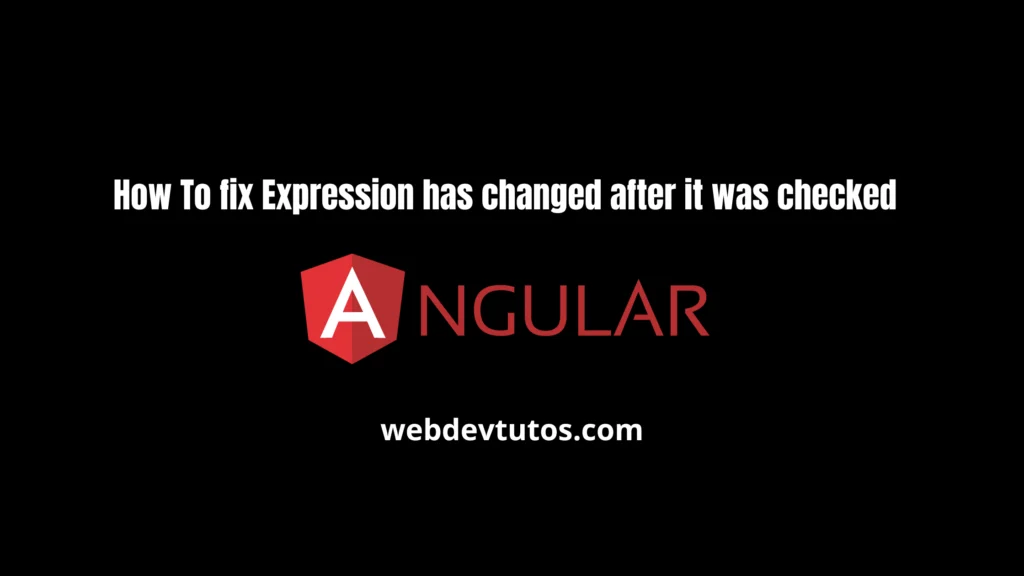
How to fix Expression has Changed After it was Checked
When you see the “Expression has changed after it was checked” error message, it means that your code is updating the view after Angular has already run its change detection cycle. There are a few ways to fix this error in Angular.
Use the ngAfterViewInit() method
This method allows you to run code after the view has been initialized. You can use this method to update any component variables or make any changes to the view. Here’s an example:
ngAfterViewInit() {
setTimeout(() => {
this.myVariable = 'new value';
});
}
Use the ChangeDetectorRef.detectChanges() method
This method triggers a change detection cycle manually. You can use this method to force Angular to check for changes in your component. Here’s an example:
constructor(private cdRef: ChangeDetectorRef) {}
ngAfterViewInit() {
this.cdRef.detectChanges();
}
Use the ngZone.run() method
This method allows you to run code outside of Angular’s zone. This can be useful if you are using a third-party library that updates the view outside of Angular. Here’s an example:
constructor(private ngZone: NgZone) {}
ngAfterViewInit() {
this.ngZone.runOutsideAngular(() => {
// Update the view using a third-party library
});
}
Use the setTimeout() method
This method delays the execution of code and allows Angular to run its change detection cycle before updating the view. Here’s an example:
ngAfterViewInit() {
setTimeout(() => {
this.myVariable = 'new value';
}, 0);
}
Conclusion
In conclusion, the “Expression has changed after it was checked” error in Angular can be solved in a few ways. By using the ngAfterViewInit() method, ChangeDetectorRef.detectChanges() method, ngZone.run() method, or setTimeout() method, you can fix this error and update your view in Angular. Remember to always check for errors in your code and try different solutions until you find the one that works for your specific case. Happy coding with our Blog!