You may work on an Angular project that does not contain a routing file, Or while creating a new Angular Project with ng new command, you forget to set the routing config. So how to do to add routing to an existing Angular project automatically without adding the file manually?
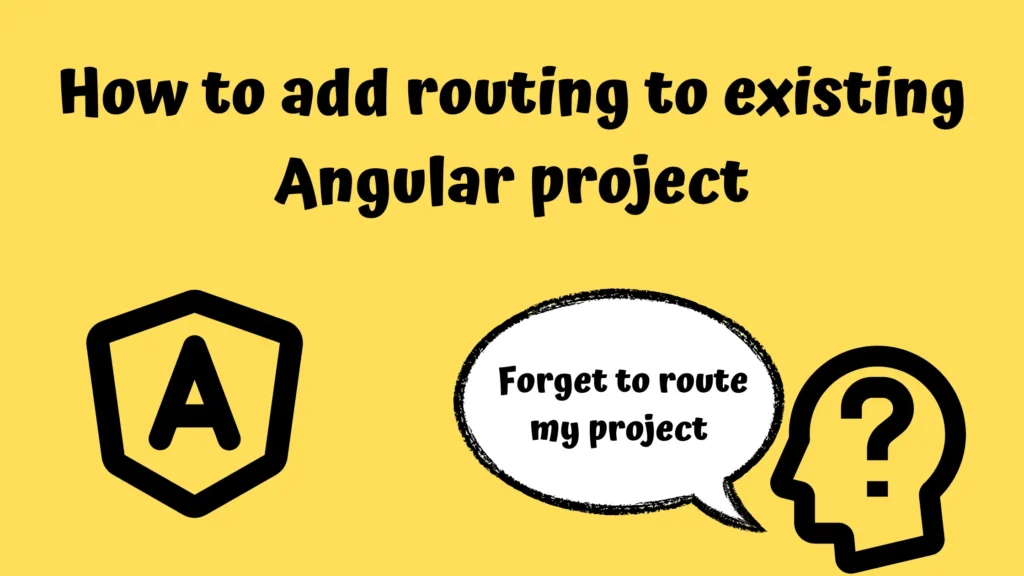
How to add routing to existing Angular project
It is simply done with a simple command line.
ng generate module app-routing --flat --module=app
This command will automatically add app-routing.module.ts file containing this code.
//app-routing.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
@NgModule({
declarations: [],
imports: [
CommonModule
]
})
export class AppRoutingModule { }
Returning to the command options :
- flat option: it will not let the generated module file be in a separate folder.
- module option: it will ensure that the generated module will be imported into the AppModule.
Now, It’s time to the Routes Array into our app-routing.module.ts. let’s see How!
//app-routing.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { RouterModule, Routes } from '@angular/router';
import { ProductListComponent } from './product-list/product-list.component';
const routes: Routes = [
{path:"products",component:ProductListComponent}
]
@NgModule({
declarations: [],
imports: [
CommonModule,
RouterModule.forRoot(routes)
],
exports: [ RouterModule ]
})
export class AppRoutingModule { }
We have finished the routing. Whenever we navigate to the /products, we will have the product list component loaded.
Conclusion
In order to add routing to an existing Angular project, we can easily type ng generate module app-routing –flat –module=app.
Follow our Content from Here.